In today’s dynamic business landscape, organizational charts are more than just static diagrams — they are essential tools for ensuring clarity, boosting productivity, and fostering better communication across teams. An effective organizational chart does more than display titles and hierarchies; it helps employees understand reporting relationships, visualize collaboration structures, and identify decision-making pathways. For instance, in a growing company with multiple departments, an organizational chart can help new hires quickly grasp who to contact for specific tasks or approvals. Similarly, in project-based work environments, dynamic organizational charts can streamline communication by showing cross-functional team structures.
Whether it’s a sales team tracking key managers and their responsibilities, or an engineering department mapping out specialized roles, having a well-organized and interactive chart makes a significant difference in operational efficiency. Recognizing this need, modern applications like AlurKerja integrate powerful organizational chart features to give teams a seamless way to visualize their structures, collaborate, and adapt to changes in real time.
But what if you want to build your own custom organizational chart feature? Whether you’re a developer looking to enhance your app or a curious learner, this guide will walk you through the steps to create an interactive organizational chart using JavaScript (or React in this example). We will use React D3 Tree library which is a React component that lets us represent hierarchical data (e.g. family trees, organizational charts, file directories) as an interactive tree graph with minimal setup, by leveraging D3’s tree
layout.
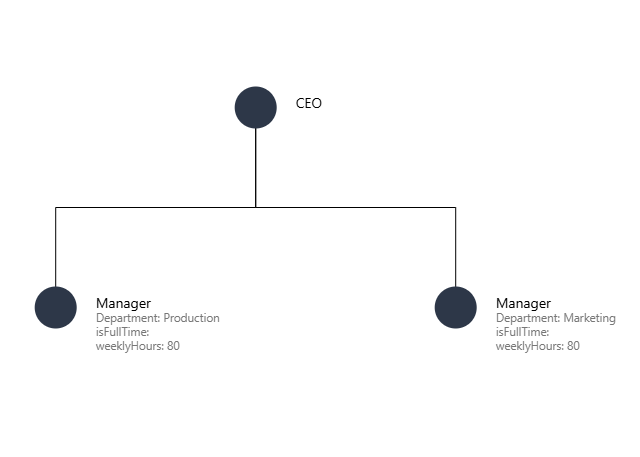
For this example, you can use new React project or integrate this into your existing React project.